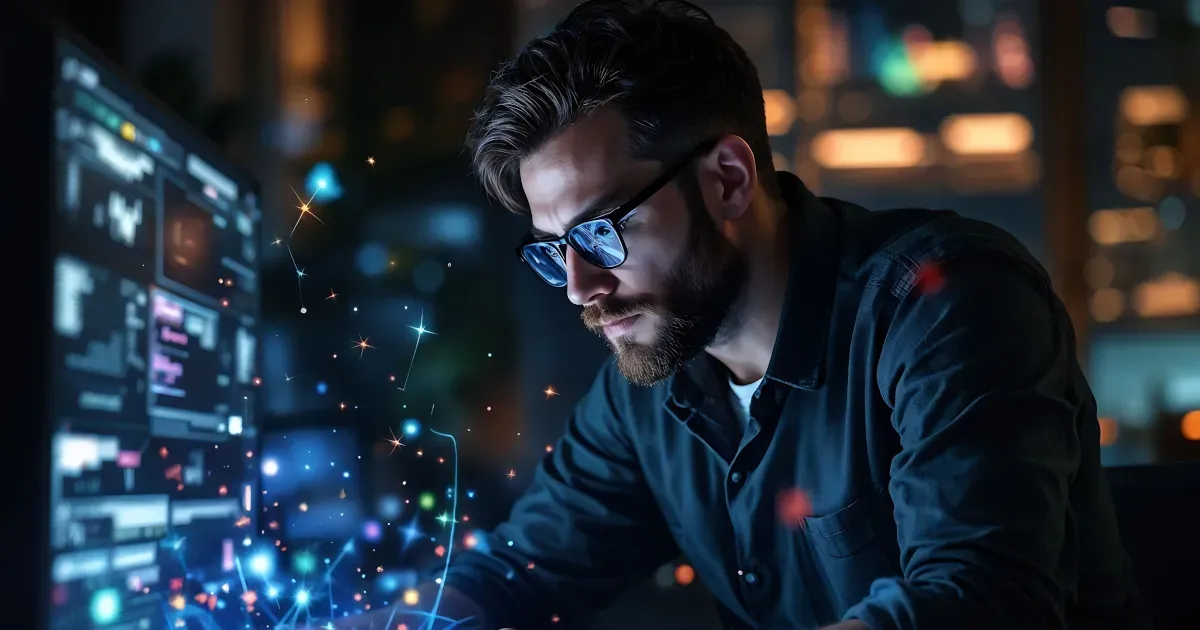
Structured Bindings: A Handy Tool for CPP
Structured Bindings: A Handy Tool for CPP
If you’re a C++ developer who appreciates writing cleaner, more readable code, then structured bindings are your liberation. This feature, introduced in C++17, liberates you from the burden of breaking down complex data structures. It’s a simple way to handle tuples or pair-like objects, letting you assign names to their components directly. No more bulky, complicated code to unpack a few variables-just relief!
Structured bindings let you deconstruct tuples, structs, or pairs much cleaner. Before C++17, you had to use std::get to retrieve elements from a tuple, which wasn’t always intuitive or clean. With structured bindings, you can declare all the parts in one line, making your code more concise and easier to understand. This not only improves the readability and maintainability of your code but can also lead to performance improvements, as it reduces the overhead of unpacking complex data structures.
Coming up, we’ll dive into structured bindings in C++, how they simplify code, and practical examples of their usage. Let’s get started!
#include <iostream>
#include <string>
struct Person {
std::string name;
int age;
bool is_employed;
};
int main() {
Person person = {"Alice", 30, true};
// Using structured bindings to extract values from the struct
auto [name, age, is_employed] = person;
std::cout << "Name: " << name << "\n";
std::cout << "Age: " << age << "\n";
std::cout << "Employed: " << (is_employed ? "Yes" : "No") << "\n";
}
In this example, we have a Person
struct with three members: name
, age
, and is_employed
. We create an instance of Person
and then use structured bindings to extract the values of its members into separate variables. Does that look cleaner to you? It sure does to me!
More Examples with Different Data Types
Structured bindings are not limited to structs; they can also be used with other data types like tuples, arrays, and pairs.
#include <iostream>
#include <tuple>
int main() {
std::tuple<std::string, int, double> data = {"Temperature", 25, 72.5};
// Using structured bindings to unpack the tuple
auto [label, count, value] = data;
std::cout << "Label: " << label << "\n";
std::cout << "Count: " << count << "\n";
std::cout << "Value: " << value << "\n";
}
Exactly the same as before, but this time with a tuple. The syntax is consistent and straightforward, making it easy to work with different data types. We have a tuple with a string, an integer, and a double, and we use structured bindings to unpack the tuple into separate variables. It’s as simple as that!
Let’s look at another example with an array:
#include <iostream>
#include <array>
#include <numeric>
int main() {
std::array<int, 5> numbers = {10, 20, 30, 40, 50};
// Using structured bindings to extract elements from the array
auto [a, b, c, d, e] = numbers;
std::cout << "a: " << a << "";
std::cout << "b: " << b << "";
std::cout << "c: " << c << "";
std::cout << "d: " << d << "";
std::cout << "e: " << e << "";
// Additional operations using the extracted elements
int sum = a + b + c + d + e;
std::cout << "Sum: " << sum << "";
int product = std::accumulate(numbers.begin(), numbers.end(), 1, std::multiplies<int>());
std::cout << "Product: " << product << "";
}
Here, we use structured bindings to extract the three elements from an std::array
. This allows us to work with individual elements directly without having to access them via indices.
#include <iostream>
#include <utility>
int main() {
std::pair<std::string, int> item = {"Apple", 50};
// Again! Using structured bindings to unpack the pair
auto [name, quantity] = item;
std::cout << "Item: " << name << "\n";
std::cout << "Quantity: " << quantity << "\n";
}
In this example, we have a std::pair
representing an item and its quantity. Principle remains the same: we use structured bindings to unpack the pair into separate variables. It’s a clean and concise way to work with pairs.
Let’s take a nested structure as an example:
#include <iostream>
#include <string>
struct Address {
std::string city;
std::string street;
};
struct Person {
std::string name;
int age;
Address address;
};
int main() {
Person person = {"Bob", 40, {"New York", "5th Avenue"}};
// Using structured bindings to extract nested values
auto [name, age, address] = person;
auto [city, street] = address;
std::cout << "Name: " << name << "\n";
std::cout << "Age: " << age << "\n";
std::cout << "City: " << city << "\n";
std::cout << "Street: " << street << "\n";
}
Why Use Structured Bindings?
From my perspective there are three main reasons to use structured bindings:
-
Structured bindings are especially useful when dealing with functions that return multiple values or containers that store data in a more complex form. They help in a few key ways:
-
Less boilerplate means fewer chances for mistakes. Instead of calling multiple
std::get<>
functions, you can use a single structured binding to extract all the values in one go. -
Structured bindings improve readability by making it immediately clear which values are being unpacked and how they relate to each other. This is especially useful when working with key-value pairs in maps.
If you’re writing C++17 or later code and want to make your functions return multiple values or are working with containers like tuples, maps, or pairs, structured bindings are a great choice. Your code will be easier to read, and it will make you look like you know what you’re doing.
So, the next time you’re dealing with complex structures, give structured bindings a shot. They’ll save you lines of code, reduce clutter, and make your programs easier to understand for both you and others.
Happy coding!