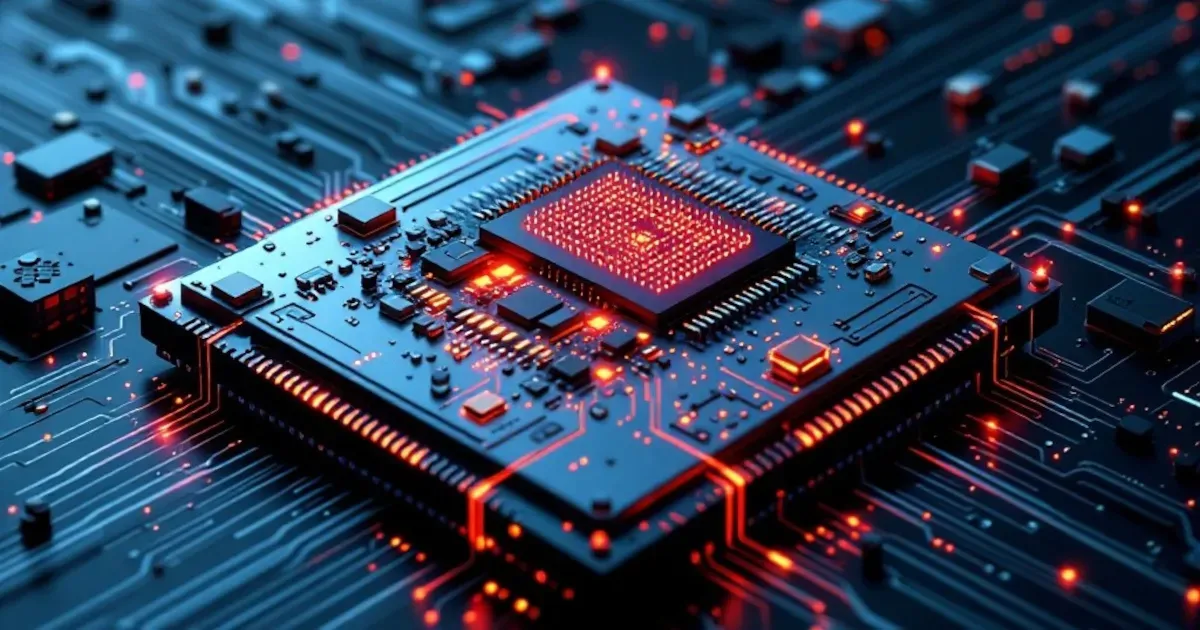
SIMD As A Gateway to High-Performance Parallel Computing
SIMD As A Gateway to High-Performance Parallel Computing
The <simd>
header introduced in C++26 represents a significant step in leveraging modern hardware’s parallel processing capabilities. However, it is important to note that features in C++26 are provisional and subject to change, as the standard evolves. SIMD, or Single Instruction Multiple Data, refers to a programming paradigm where a single operation can be applied simultaneously to multiple pieces of data. For example, instead of processing each pixel in an image one at a time, SIMD allows entire rows or sections of the image to be handled in parallel, dramatically reducing computation time. This capability is critical for tasks that require high computational throughput, such as running large-scale numerical simulations, manipulating high-resolution images, performing extensive data analysis, or maintaining responsiveness in real-time systems like video games or financial platforms. By streamlining operations across multiple data points at once, SIMD delivers significant speed improvements that can transform how developers approach performance-intensive applications. The code examples provided in this article are conceptual demonstrations, designed to showcase potential use cases of <simd>
rather than production-ready implementations. Proper alignment, error handling, and validation should be considered when integrating such solutions into real-world applications.
By abstracting low-level hardware details, <simd>
allows developers to write clean, portable code while achieving remarkable speedups. Its high-level interface reduces the need for platform-specific intrinsics or assembly code, such as those provided by compiler intrinsics (e.g., Intel’s SSE and AVX), platform-specific APIs (like Apple’s Accelerate framework), or third-party libraries (e.g., Eigen and Boost.SIMD). This broadens its appeal to developers looking for a unified and standardized approach to SIMD programming. This article explores the practical applications of <simd>
with two real-world examples and highlights its benefits and considerations.
To understand the practical implications of <simd>
, let’s explore two real-world examples. These examples demonstrate how SIMD can drastically improve the performance of computationally intensive operations.
Example 1: Transactional System
High-frequency financial systems must process and validate thousands of transactions in real-time. SIMD allows developers to parallelize these repetitive operations efficiently. Consider a system that calculates a fraud detection score for each transaction based on the transaction amount, frequency, and account history.
#if __has_include(<simd>)
#include <simd>
#define HAS_STD_SIMD 1
#else
#define HAS_STD_SIMD 0
#endif
#include <vector>
#include <iostream>
void processTransactions(const std::vector<float>& transactionAmounts,
const std::vector<float>& transactionFrequencies,
const std::vector<float>& accountHistories,
std::vector<float>& fraudScores) {
size_t size = transactionAmounts.size();
fraudScores.resize(size);
#if HAS_STD_SIMD
using namespace std;
for (size_t i = 0; i < size; i += simd<float>::size()) {
simd<float> amounts = simd<float>(&transactionAmounts[i], element_aligned);
simd<float> frequencies = simd<float>(&transactionFrequencies[i], element_aligned);
simd<float> histories = simd<float>(&accountHistories[i], element_aligned);
simd<float> scores = amounts * 0.5f + frequencies * 0.3f + histories * 0.2f;
scores.copy_to(&fraudScores[i], element_aligned);
}
#else
for (size_t i = 0; i < size; ++i) {
fraudScores[i] = transactionAmounts[i] * 0.5f +
transactionFrequencies[i] * 0.3f +
accountHistories[i] * 0.2f;
}
#endif
}
int main() {
#if HAS_STD_SIMD
std::cout << "Using std::simd implementation for transaction processing\n";
#else
std::cout << "Using scalar implementation for transaction processing\n";
#endif
std::vector<float> transactionAmounts = {100.0f, 200.0f, 150.0f, 300.0f};
std::vector<float> transactionFrequencies = {1.0f, 0.5f, 0.8f, 0.2f};
std::vector<float> accountHistories = {0.9f, 0.7f, 0.5f, 0.3f};
std::vector<float> fraudScores;
processTransactions(transactionAmounts, transactionFrequencies, accountHistories, fraudScores);
for (float score : fraudScores) {
std::cout << "Fraud Score: " << score << std::endl;
}
return 0;
}
The preprocessor first detects if <simd>
is available and accordingly selects the most suitable implementation. SIMD then enables vectorized operations, allowing multiple transactions to be processed simultaneously, significantly reducing latency. In the absence of SIMD support, a scalar fallback ensures portability and compatibility across all systems. This combination ensures efficiency, particularly at scale, where systems processing millions of transactions daily can benefit from the resource savings and speedup provided by SIMD.
Example 2: Physics Simulation
In gaming, physics simulations involving particle interactions are computationally expensive. Each frame requires updating particle positions and handling collisions. SIMD can optimize this by processing particle attributes in parallel.
#include <iostream>
#include <vector>
#include <cmath>
#if __has_include(<simd>)
#include <simd>
#define HAS_STD_SIMD 1
#else
#define HAS_STD_SIMD 0
#endif
void simulateParticles(const std::vector<float>& positionsX,
const std::vector<float>& positionsY,
const std::vector<float>& velocitiesX,
const std::vector<float>& velocitiesY,
float deltaTime,
std::vector<float>& updatedPositionsX,
std::vector<float>& updatedPositionsY) {
size_t size = positionsX.size();
updatedPositionsX.resize(size);
updatedPositionsY.resize(size);
#if HAS_STD_SIMD
using namespace std;
for (size_t i = 0; i < size; i += simd<float>::size()) {
simd<float> posX = simd<float>(&positionsX[i], element_aligned);
simd<float> posY = simd<float>(&positionsY[i], element_aligned);
simd<float> velX = simd<float>(&velocitiesX[i], element_aligned);
simd<float> velY = simd<float>(&velocitiesY[i], element_aligned);
simd<float> newPosX = posX + velX * deltaTime;
simd<float> newPosY = posY + velY * deltaTime;
newPosX.copy_to(&updatedPositionsX[i], element_aligned);
newPosY.copy_to(&updatedPositionsY[i], element_aligned);
}
#else
for (size_t i = 0; i < size; ++i) {
updatedPositionsX[i] = positionsX[i] + velocitiesX[i] * deltaTime;
updatedPositionsY[i] = positionsY[i] + velocitiesY[i] * deltaTime;
}
#endif
}
int main() {
#if HAS_STD_SIMD
std::cout << "Using std::simd implementation for particle simulation\n";
#else
std::cout << "Using scalar implementation for particle simulation\n";
#endif
std::vector<float> positionsX = {1.0f, 2.0f, 3.0f, 4.0f};
std::vector<float> positionsY = {1.0f, 2.0f, 3.0f, 4.0f};
std::vector<float> velocitiesX = {0.1f, 0.2f, 0.3f, 0.4f};
std::vector<float> velocitiesY = {0.1f, 0.2f, 0.3f, 0.4f};
std::vector<float> updatedPositionsX;
std::vector<float> updatedPositionsY;
float deltaTime = 0.016f; // Simulating 60 FPS
simulateParticles(positionsX, positionsY, velocitiesX, velocitiesY, deltaTime, updatedPositionsX, updatedPositionsY);
for (size_t i = 0; i < updatedPositionsX.size(); ++i) {
std::cout << "Particle " << i << " new position: ("
<< updatedPositionsX[i] << ", "
<< updatedPositionsY[i] << ")" << std::endl;
}
return 0;
}
SIMD efficiently updates positions and velocities in parallel, ensuring high frame rates and smooth performance. This optimization is particularly crucial for real-time physics simulations commonly found in games and VR applications, where responsiveness is key. Additionally, careful attention must be given to proper alignment of memory and error handling, as unaligned or invalid data can cause performance penalties or unexpected behavior. As the number of particles in the simulation grows, SIMD’s scalability allows the system to handle increasing computational demands without significant performance degradation, maintaining the simulation’s interactivity and realism.
Beyond these examples, the <simd>
header enables efficient solutions for numerous other domains. In image processing, it facilitates the parallelization of pixel-level operations such as filtering and transformations, leading to significant speedups. In machine learning, SIMD accelerates tensor computations and neural network inference, optimizing performance for training and inference tasks. Additionally, in scientific computing, it enhances the performance of simulations and data analysis pipelines, making large-scale computations more efficient and scalable.
The <simd>
header in C++26 stands out as a practical tool that empowers developers to take full advantage of modern hardware’s parallel processing capabilities. It simplifies the process of optimizing tasks such as financial computations, gaming simulations, and other resource-intensive operations. By using <simd>
, developers can achieve significant performance improvements while maintaining clean and portable code. This makes <simd>
an invaluable resource for programmers looking to balance efficiency with code clarity in performance-critical applications.