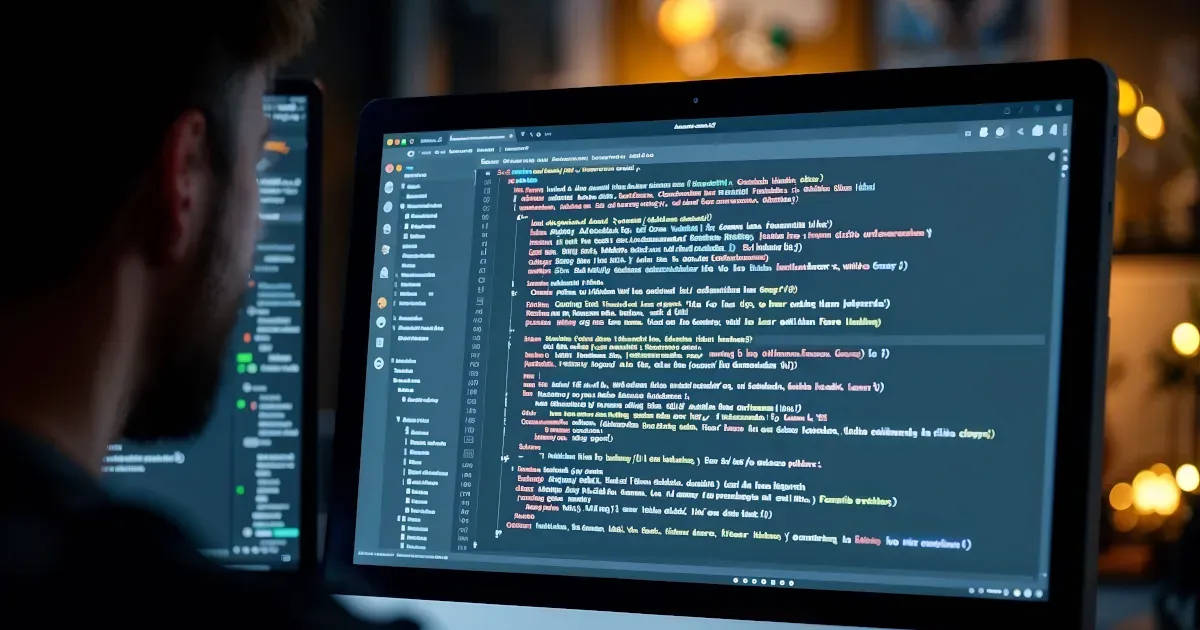
Rethinking Strings with std::string_view
Rethinking Strings with std::string_view
When C++17 introduced std::string_view
, it felt like discovering a secret tool you didn’t know you needed. At first glance, it seems simple,a way to look at a string without owning it. But under the hood, it’s much more. It represents a fundamental shift in how we think about performance, efficiency, and the true cost of convenience in code. By challenging the default assumptions developers hold about strings, it forces a reevaluation of practices that often prioritize ease of use over measurable performance gains.
To truly understand its importance, consider how it integrates into the broader ecosystem of C++ tools. std::string_view
transcends its role as a utility for strings,it’s a cornerstone of a more deliberate programming paradigm, one where the boundaries between ownership and access are well-defined. This represents a departure from the hidden costs of implicit memory management, moving instead toward an approach that champions explicit, transparent resource usage. The ripple effects of this shift are reshaping how developers solve problems in modern C++.
The Problem with std::string
As C++ programmers, we all relied heavily on std::string
. It’s robust, handles memory for you, and generally “just works.” But with every convenience, there’s a cost. Every copy operation means allocating, copying, and sometimes deallocating memory. For high-performance code, these hidden costs stack up quickly.
Enter std::string_view
. Instead of owning data, it merely observes it. Consider it as borrowing a library book,you don’t own it. However, you can still read it without worrying about ownership overhead.
This distinction between owning and observing is subtle but powerful. Functions that demand multiple overloads for std::string
, const char*
, or even string literals suddenly become simpler. With std::string_view
, you write one function that works for all these cases. It’s more than just syntactic sugar,it’s a fundamental change in how we think about strings.
Why std::string_view
Matters
The best tools solve specific problems. For std::string_view
, there are two primary ones: unnecessary copies and overly complex APIs.
Consider a logging function. You want it to handle both std::string
and const char*
. Traditionally, you’d either write overloads or convert one type into the other. Both approaches have downsides,either more code or unnecessary copies. With std::string_view
, these problems disappear and pave the way for cleaner, more efficient design. For instance, imagine a situation where you’re processing log entries in a performance-critical application. Each entry might come from different sources,some dynamically allocated strings, others constant literals, or even substrings from a larger buffer. By standardizing on std::string_view
, you reduce complexity in your API while avoiding the overhead of unnecessary memory allocations. This simplicity doesn’t just save time for developers; it directly impacts runtime performance by minimizing redundant operations. By enabling seamless integration of various string-like sources, std::string_view
fosters an environment where your design is not only concise but also highly modular. The result is not just a better-performing system, but also one that’s easier to maintain, extend, and scale to future needs.
void logMessage(std::string_view message) {
std::cout << "Log: " << message << '\n';
}
This isn’t just about elegance. By passing a std::string_view
, you avoid copying large strings unnecessarily. For high-performance systems, the savings are immediate and measurable. For example, consider processing a large dataset of strings in a logging system. Using std::string_view
to pass the strings eliminates unnecessary copies, reducing memory usage and execution time significantly. In benchmarks, systems leveraging std::string_view
often demonstrate lower memory overhead and faster processing, particularly when handling thousands of operations per second.
What Makes std::string_view
Special?
At its core, std::string_view
is a lightweight structure. It holds a pointer to the data and its size, nothing more. This design makes it efficient and compact. Unlike raw pointers, it provides bounds-checked operations and doesn’t assume null termination, which can lead to pitfalls when code relies on C-style string functions requiring a null-terminator. This flexibility is particularly useful when dealing with non-null-terminated buffers or strings containing embedded null characters, but it also presents a challenge for interoperability with C-style string functions, which rely on null termination. The lack of a guaranteed null-terminator can lead to subtle bugs when code assumes it can safely use such functions with std::string_view
. Developers must explicitly handle these cases, ensuring that a null-terminated version of the data is available if needed.
std::string_view sv("Hello\0World", 11);
std::cout << sv.size(); // Outputs 11
But the real magic lies in how it integrates with modern C++ features. For instance, std::string_view
supports constexpr
, allowing string computations at compile time:
constexpr std::string_view sv = "Compile-time string";
constexpr auto len = sv.size(); // Evaluated at compile time
This opens doors for template metaprogramming and other advanced compile-time techniques.
The Risks of std::string_view
Of course, not every tool is foolproof. The primary pitfall with std::string_view
is dangling references, making it essential to ensure that the view does not outlive the data it references. This issue is critical and one of the most significant considerations when using std::string_view
in your code. Since it doesn’t own the data, it’s easy to accidentally reference something that no longer exists, leading to potential dangling references. Developers can safeguard against this by employing lifetime management strategies, such as ensuring that the data outlives the std::string_view
or by avoiding temporary objects altogether. For example, one practical approach is to use objects with well-defined lifetimes, like class members or global variables, to back the std::string_view
. These strategies significantly reduce the risk of undefined behavior and make std::string_view
safer to use in complex applications.
std::string_view dangerous() {
std::string temp = "Temporary";
return std::string_view(temp); // Undefined behavior
}
Another limitation is immutability. std::string_view
provides read-only access. If you need to modify the data, you’ll have to convert it back to a modifiable type like std::string
.
When Not to Use std::string_view
Despite its many benefits, there are scenarios where std::string_view
is not the best choice:
If your code needs to modify the underlying data, std::string_view
isn’t the right tool. It’s read-only by design. For any situation where changes to the string are necessary, a mutable type like std::string
should be used instead.
Lifetime uncertainty is another major limitation. Using std::string_view
when there’s a chance of accessing invalid memory is risky. This includes scenarios where temporary objects are involved or where the underlying data’s lifespan is unclear. The risk of dangling references cannot be overstated, and it requires careful management to avoid undefined behavior.
Multi-threaded code introduces further complications. If there’s any possibility that another thread might reallocate or alter the referenced data, using std::string_view
can lead to unpredictable results. Ensuring thread safety with proper synchronization or copying the data might be necessary to maintain stability and correctness in such environments.
By understanding these limitations, developers can make informed choices about integrating std::string_view
into their code.
Transforming APIs
One of the most immediate benefits of std::string_view
is how it simplifies APIs. Functions that once required multiple overloads for different string types can now use a single interface. For example:
void processString(std::string_view sv);
This design not only reduces boilerplate but also encourages cleaner, more maintainable codebases. For large projects, these small changes compound into significant improvements in readability and performance.
Advice for Adopting in Existing Code
Refactoring existing code to use std::string_view
can significantly improve performance and clarity. However, it requires careful consideration to avoid common pitfalls. When replacing std::string
with std::string_view
, ensure that the data being referenced outlives the view. This might involve auditing functions and ensuring that temporaries or dynamically allocated memory aren’t prematurely destroyed.
A good starting point is to identify functions that currently take std::string
parameters and assess whether they could use std::string_view
. By switching to std::string_view
, you reduce unnecessary copies and potentially improve performance. For example:
void oldFunction(const std::string &input) {
// Existing code
}
void newFunction(std::string_view input) {
// Refactored code
}
To ensure safety, consider pairing std::string_view
with well-defined lifetime management strategies. For instance, avoid passing views of temporary objects and prefer referencing objects with predictable lifetimes, such as those allocated on the stack or with static storage duration. Testing and careful review are crucial during this transition to catch and address potential dangling references. Additionally, employing tools like static analyzers can help identify potential risks early in the refactoring process. Using std::string_view
safely often requires a deeper understanding of how data lifetimes are managed in your application, making thorough documentation and team training essential. These precautions will not only streamline the adoption process but also enhance the robustness of your codebase.
Beyond Strings: A Broader Trend in C++
std::string_view
is part of a larger movement in modern C++: separating ownership from access. You see the same philosophy in std::span
for arrays, std::optional
for nullable values, and the ranges library introduced in C++20. This larger trend also includes tools like std::unique_ptr
and std::shared_ptr
, which make ownership explicit in memory management, and concepts like std::variant
, which allow type-safe alternatives to unions. For example, consider a scenario where std::unique_ptr
interacts with std::string_view
to manage ownership explicitly while passing string data efficiently:
#include <iostream>
#include <memory>
#include <string_view>
void printView(std::string_view sv) {
std::cout << "View: " << sv << '\n';
}
int main() {
auto str = std::make_unique<std::string>("Managed String");
printView(*str);
return 0;
}
In this example, std::unique_ptr
owns the string, ensuring its lifetime is managed correctly, while std::string_view
allows efficient, read-only access. Each of these features encourages developers to write cleaner, safer, and more efficient code while minimizing ambiguity about who owns what.
Why std::string_view
is Worth Mastering
Some tools subtly change how you write code. std::string_view
is not just about performance,it’s a reminder to think critically about how we manage resources. It forces us to question whether ownership is necessary and encourages better API design.
If you haven’t used std::string_view
yet, start with something simple. Refactor an overloaded function to take a std::string_view
. Use it in a high-frequency loop where string operations dominate. You’ll notice the difference quickly.
Modern C++ is full of tools that challenge assumptions. std::string_view
stands out not just for what it does, but for how it reshapes our thinking. It’s not just another tool,it’s a new way to write code.
References
-
C++ reference on std::string_view (available at https://en.cppreference.com/w/cpp/string/basic_string_view) [Accessed: 02.01.2025].
-
Performance of
std::string_view
vsstd::string
from C++17 (available at https://www.cppstories.com/2018/07/string-view-perf) [Accessed: 02.01.2025]. -
Performance Analysis of C++17’s
std::string_view
(available at https://codalogic.com/blog/2021/11/18/Performance-Analysis-of-C%2B%2B17%27s-std__string_view) [Accessed: 02.01.2025]. -
C++17 – Avoid Copying with
std::string_view
(available at https://www.modernescpp.com/index.php/c-17-avoid-copying-with-std-string-view) [Accessed: 02.01.2025]. -
C++
std::string_view
for Better Performance: An Example Use Case (available at https://www.nextptr.com/tutorial/ta1217154594/cplusplus-stdstring_view-for-better-performance-an-example-use-case) [Accessed: 02.01.2025]. -
Effortless Performance Improvements in C++:
std::string_view
(available at https://julien.jorge.st/posts/en/effortless-performance-improvements-in-cpp-std-string_view) [Accessed: 02.01.2025].