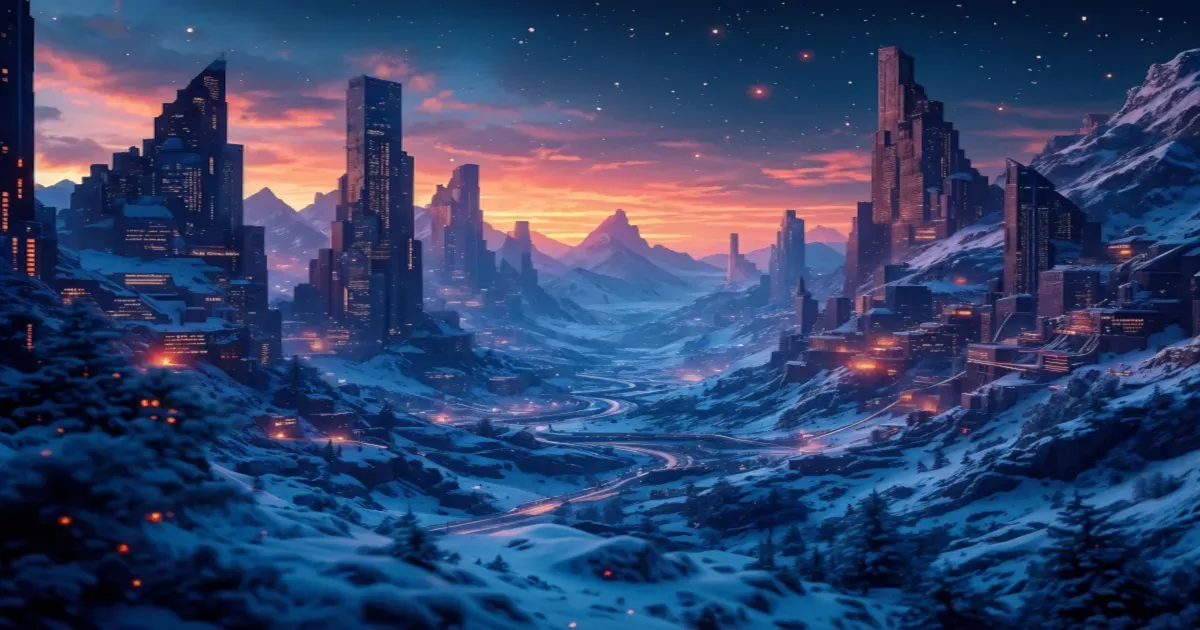
Understanding std::byteswap in C++
Understanding std::byteswap
in C++
The introduction of std::byteswap in C++23 marks a significant addition to the Standard Library, catering to developers working with data that requires endianness manipulation.
Imagine the hexadecimal value 0x12345678
stored in a 32-bit integer. In little-endian, it is stored as 42 71 34 11
, but in big-endian architecture, it is stored as 11 34 71 42
. Such differences often require conversion when sharing data between systems, and std::byteswap
simplifies this task. Endianness, the order in which bytes are stored and processed, can be a critical aspect of system programming, networking, and file I/O. My today’s blog article will dive into the purpose, usage, and practical applications of std::byteswap
.
What is std::byteswap
?
std::byteswap
is a function template provided by the C++ Standard Library in the <numeric>
header. It facilitates the byte-swapping operation, reversing the order of bytes in an integer value. This operation is often necessary when converting data between big-endian and little-endian systems.
The function’s prototype looks like this:
namespace std {
template <class IntegerType>
constexpr IntegerType byteswap(IntegerType value) noexcept;
}
Short explanation:
IntegerType
is the type of the integer to swap, which must be an integral type.- The
value
parameter is the integer value whose bytes are to be swapped. - The function returns the byte-swapped equivalent of the input value.
- The
IntegerType
must be an integral type, including signed and unsigned variants. Using any kind of non-integral types will result in a compile-time error.
Endianness issues often arise when working across systems with differing architectures. In networking, data transmitted over networks usually follows a big-endian convention (network byte order), while many modern CPUs use little-endian order. Binary file formats often mandate specific byte orders, requiring conversions during read/write operations. Cross-platform applications that share data between platforms need a reliable way to adjust byte order.
Before C++23, developers had to rely on platform-specific or custom implementations to handle byte-swapping. std::byteswap
standardizes this operation, providing a portable and consistent solution.
So how to use it? Here is a very standard and basic example showing you how
#include <iostream> // Header for IO stream functions
#include <numeric> // Include this header for std::byteswap
int main() {
// Define an original 32-bit integer value
uint32_t original = 0x12345678;
// Swap the byte order
uint32_t swapped = std::byteswap(original);
// Print the original value in hexadecimal
std::cout << "Original: 0x" << std::hex << original << "\n";
// Print the swapped value in hexadecimal
std::cout << "Swapped: 0x" << std::hex << swapped << "\n";
// Exit the program
return 0;
}
The example demonstrates swapping the bytes of a 32-bit unsigned integer. The result shows how the byte order is reversed. It will give you following output:
Original: 0x12345678
Swapped: 0x78563412
Practical Examples
For network programming, byte-swapping is crucial when sending and receiving data over the network. Network protocols often specify big-endian byte order, requiring conversion when working with little-endian systems. The std::byteswap
function simplifies this process, ensuring that data is correctly formatted for network transmission.
Here is an example demonstrating how std::byteswap
can be used in network programming:
#include <cstdint>
#include <numeric>
uint32_t to_network_order(uint32_t host_value) {
return std::byteswap(host_value);
}
uint32_t to_host_order(uint32_t network_value) {
return std::byteswap(network_value);
}
The to_network_order
function converts a host value to network byte order, while to_host_order
converts a network value to host byte order. These functions are essential for ensuring that data is correctly formatted for network communication.
Considerations
While std::byteswap
is versatile, it only operates on integral types. For example, it cannot directly handle complex data structures like struct or class objects, requiring developers to manually apply byte-swapping to individual fields. Additionally, it does not account for endianness in floating-point numbers, which often have different memory layouts across architectures.
For more complex structures, manual handling or serialization techniques are required to manage byte order. Additionally, endianness handling at a broader level may necessitate combining std::byteswap
with other utilities such as std::endian
.
A word about performance: std::byteswap
is implemented using compiler intrinsics where available, ensuring optimal performance. On modern architectures, byte-swapping instructions are highly efficient. Additionally, std::byteswap
is constexpr
, allowing compile-time evaluations when used with constant expressions.
A Real Helper in C++23
The introduction of std::byteswap
in C++23 is a significant step towards standardizing endianness manipulation. It provides a portable and efficient solution for byte-swapping operations, simplifying tasks that were previously platform-dependent or required custom implementations. Endianness issues are common, but std::byteswap
makes your life easier when working with data across different systems.
References
- C++ reference on std::byteswap (available at https://en.cppreference.com/w/cpp/numeric/byteswap) [Accessed: 17.01.2025].
- C++23 bitwise operations (available at https://www.sandordargo.com/blog/2024/01/17/cpp23-bitwise-operations) [Accessed: 17.01.2025].
- Three new utility functions in C++23 (available at https://mariusbancila.ro/blog/2022/11/08/three-new-utility-functions-in-cpp23/) [Accessed: 17.01.2025].
- C++ forum discussion on general topics (available at https://cplusplus.com/forum/general/177315/) [Accessed: 17.01.2025].
- Documentation on std::byteswap (available at https://runebook.dev/en/docs/cpp/numeric/byteswap) [Accessed: 17.01.2025].